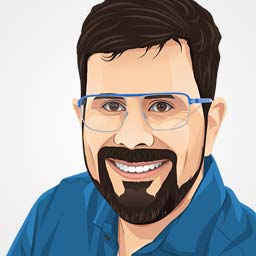
Is there one best practice for Vue js Icon Component?
There are several best practices for integrating and using icons in your single or multi page Vue js application. You can use an svg sprite, an image sprite or even an icon font.
All these solutions are great and useful but maybe you want to have more control over the icons you are using. Maybe you want to add SVG code to your source code or you want to use icons with different viewboxes. Therefore I think this Tutorial will be a great inspiration for your new Base Icon Component.
What is a Base Component in VueJS
According to the official Vue js style guide you should name a component a base component if it is unique to your application and you are using it in many places inside your app. You can name it like “BaseIcon.vue” or every other name starting with “Base”.
Start using the best practice Icon Component
First step is to go to the component on BaseIcon.vue @GitHub , copy the code and save it in a new file. You can use the component right after importing it into one of your views or other components. The usage is very simple: just add the component to your template and specify the icon you want to use - in this case “angleRight”.
More base component properties
By using the property “fade” you can define if the icon will by faded in after loading. To set “fade” true or false you can use regular string no need for :fade to get and boolean. “aspect” property defines the preserveAspectRatio attribute inside the SVG code. With “none” you can set the icon to be scaled without preserving the aspect ratio.
<template>
<div>
<div class="button text-green-600">
<BaseIcon name="angleRight" />
</div>
<div class="button text-green-600">
<BaseIcon name="angleLeft" />
</div>
</div>
</template>
<script>
import BaseIcon from '@/components/BaseIcon'
export default {
name: 'NameOfTheComponent',
components: { BaseIcon }
}
</script>
The second step of this Tutorial is as simple as the first one. Update the path definitions in the constant named “icons”. Delete every icon you don’t need and add new ones.
const icons = {
leftRight: {
viewbox: '0 0 512 512',
path: [
{
path:
'M51.038 239.05l136-136a23.9 23.9 0 0 1 33.9 0l22.6 22.6a23.9 23.9 0 0 1 0 33.9l-96.3 96.5 96.4 96.4a23.9 23.9 0 0 1 0 33.9l-22.6 22.7a23.9 23.9 0 0 1-33.9 0l-136-136a23.932 23.932 0 0 1-.1-34z',
fill: '#00b71c',
},
{
path:
'M460.55 273.05l-136 136a23.9 23.9 0 0 1-33.9 0l-22.6-22.6a23.9 23.9 0 0 1 0-33.9l96.4-96.4-96.4-96.4a23.9 23.9 0 0 1 0-33.9l22.5-22.8a23.9 23.9 0 0 1 33.9 0l136 136a23.932 23.932 0 0 1 .1 34z',
fill: '#ba1919',
},
],
},
angleRight: {
viewbox: '0 0 256 512',
path:
'M224.3 273l-136 136c-9.4 9.4-24.6 9.4-33.9 0l-22.6-22.6c-9.4-9.4-9.4-24.6 0-33.9l96.4-96.4-96.4-96.4c-9.4-9.4-9.4-24.6 0-33.9L54.3 103c9.4-9.4 24.6-9.4 33.9 0l136 136c9.5 9.4 9.5 24.6.1 34z',
},
angleLeft: {
viewbox: '0 0 256 512',
path:
'M31.7 239l136-136c9.4-9.4 24.6-9.4 33.9 0l22.6 22.6c9.4 9.4 9.4 24.6 0 33.9L127.9 256l96.4 96.4c9.4 9.4 9.4 24.6 0 33.9L201.7 409c-9.4 9.4-24.6 9.4-33.9 0l-136-136c-9.5-9.4-9.5-24.6-.1-34z',
}
}
The perfect SVG path handling
SVG files can sometimes be very confusing. Having so many transitions on one single path makes a svg file usable as an image but not for a simple understanding.
SVG files can sometimes be very confusing. For example, you can have several transitions on one single path item. This makes a SVG file although usable as an image but not easy to understand. The best way is to recalculate the path based on the used transitions and generate a minified version of the SVG path for the usage inside your VueJS Base Icon Component. A great online tool you can use for this recalculation is the SVG optimiser by Peter Collingridge or this SVG optimizer . Copy the SVG source code, paste it into the text area and “voila” you have your cleaned version of the SVG. I love it that Peter added a preview to the optimizer so you can check if there are any issues with the minified code.
Some additional tips for svg icon handling in this Tutorial
- Try to reduce the amount of different viewboxes
- Optimize every SVG code you use
- Use tools like Adobe XD, Illustrator or Figma for rearranging you SVG icons
- There is a great plugin for Adobe XD called “ Copy SVG Code ”. So you can copy the svg code so easily I love it.
Fill color in the base icon component
To change the color of the icon you can use fill in the icon object. If you don’t define it inside the object “fill-current” will be added automatically to the icon and you can use Tailwind CSS to define the color by using the suitable text color e.g. text-orange-300.
<style lang="postcss" scoped>
.icon {
@apply fill-current;
}
</style>
For the styles inside the VueJS component I prefer using BEM to be more structured. To get the most out of Tailwind CSS and Purge CSS you should add “postcss” inside the “lang” property. This makes it easier to use the Tailwind CSS directives inside the scoped styles and don#t forget to use Purge CSS whitespace comments to make sure that all classes inside the icon styles aren’t cleaned up.
Things I want to improve in this Vue js Base Component
Make the component purge css friendlyAdd multi color support to the Base ComponentMulti path supportMulticolor supportAuto animation on load optionalDefine aspect ratio of the SVG- Optimize loading of an bigger Icon Component with Webpack
- Auto generate and auto optimize SVG code
Best practice Vue JS and Tailwind CSS boilerplate
The icon base component is part of my Vue js and Tailwind CSS boilerplate which includes more best practice for navigation, notifications, the use of the Vuex store or how to define plugins in Tailwind CSS and an effective way to enhance it - you can find the repository @Github .
All links in a practical list
More articles
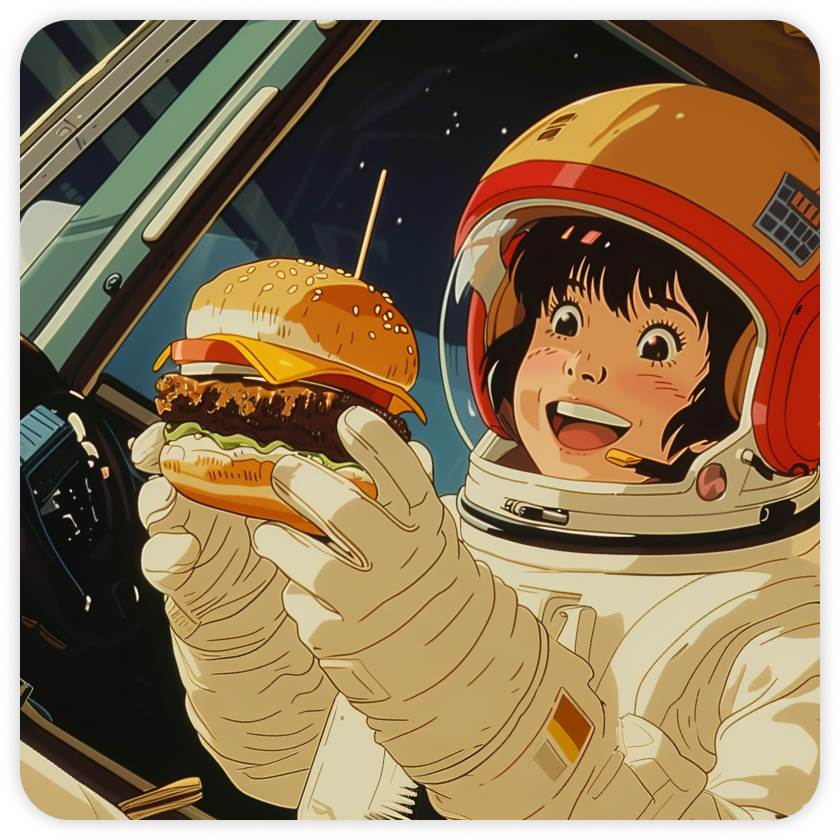
Conversational Design - The Art of Conversation
Welcome, curious minds and innovative thinkers! Today, we’re embarking on an exhilarating journey into the heart of conversational design, a realm where words become the keystrokes of …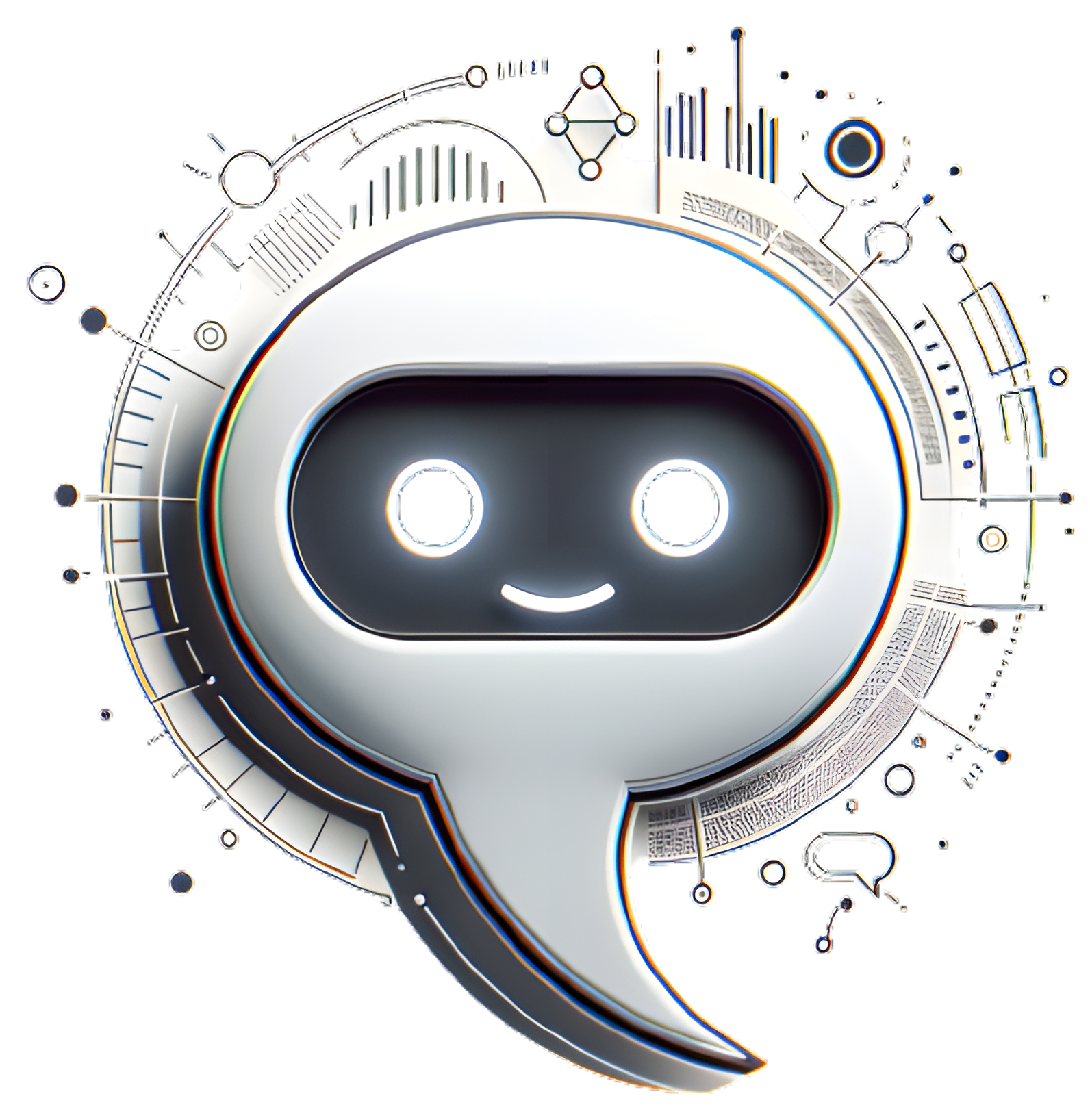
Passion, Tech, and the Conversational AI Journey!
Hello, passionate tech enthusiasts! Today, I’d love to draw a parallel between the thrilling world of Tailwind CSS and Conversational AI. Two seemingly unrelated subjects, but bear …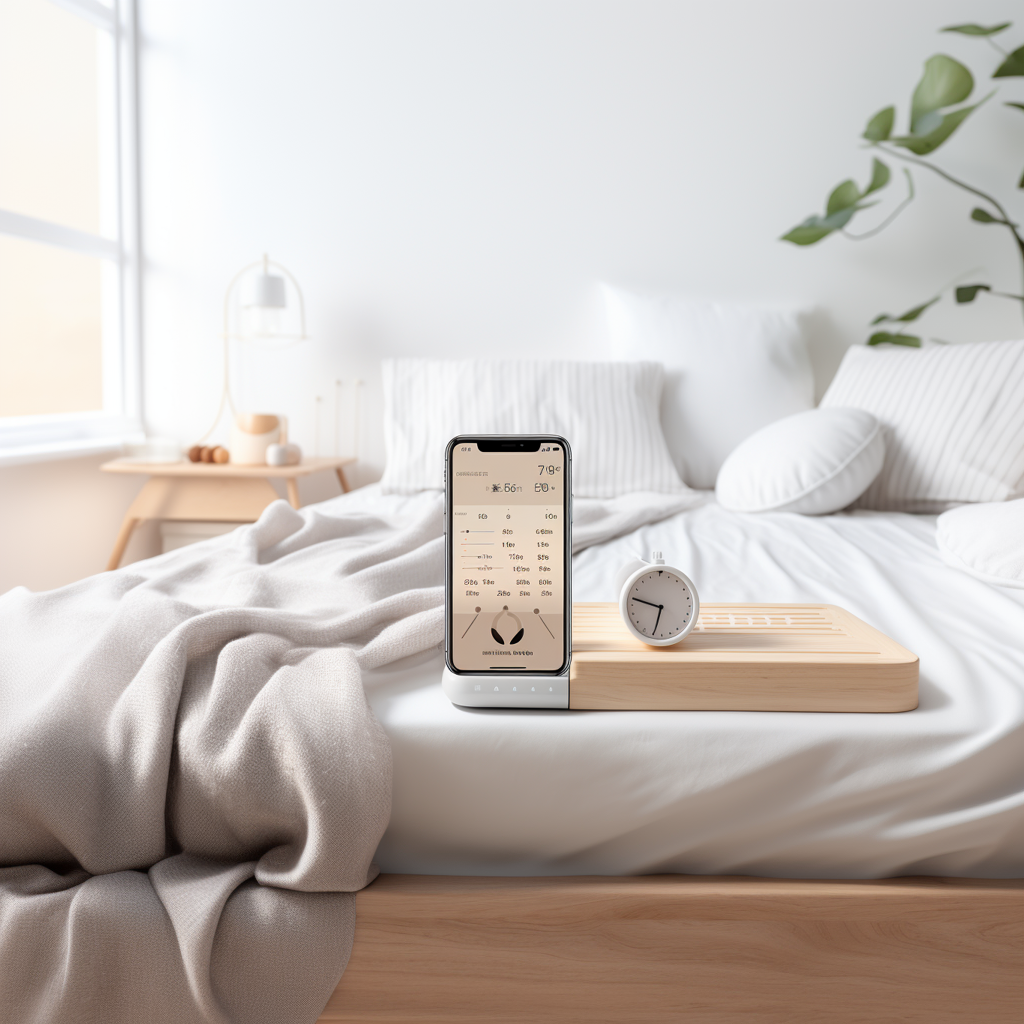